- Assembly Commands Cheat Sheet Download
- Assembly Code Cheat Sheet
- Arm Assembly Cheat Sheet
- X86 Cheat Sheet
- Assembly Commands Cheat Sheet 2020
- Arm Assembly Instructions Cheat Sheet
The cheat sheet is intended for 32-bit Windows programming with FASM. One A4 page contains almost all general-purpose x86 instructions (except FPU, MMX and SSE instructions).
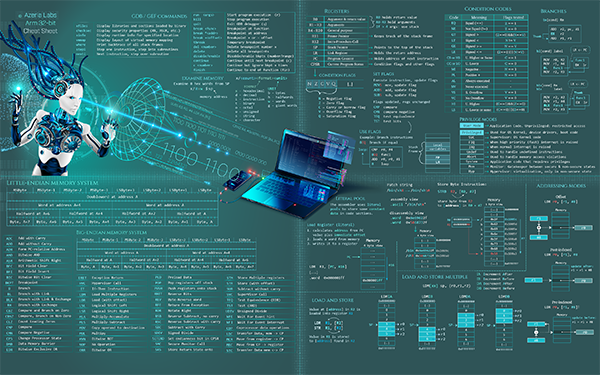
What is included
You will find various kinds of moves (MOV, CMOV, XCHG), arithmetical (ADD, SUB, MUL, DIV) and logical (AND, OR, XOR, NOT) instructions here. Several charts illustrate shifts (SHL/SHR, ROL/ROR, RCL/RCR) and stack frames. Code samples for typical high-level language constructs (if conditions, while and for loops, switches, function calls) are shown. Also included are quick references for RDTSC and CPUID instructions, description of string operations such as REP MOVSB, some code patterns for branchless conditions, a list of registers that should be saved in functions, and a lot of other useful stuff.
The C Cheat Sheet An Introduction to Programming in C Revision 1.0 September 2000 Andrew Sterian Padnos School of Engineering Grand Valley State University. The block size is the default view size for radare. All commands will work with this constraint, but you can always temporally change the block size just giving a numeric argument to the print commands for example (px 20) b size: Change block size JSON Output. Most of commands such as (i)nfo and (p)rint commands accept a j to print their output.
The idea is to put all reference information about x86 assembly language on the one page. Some rarely-used instructions such as LDS, BOUNDS or AAA are skipped.
Notation
The cheat sheet use common notation for operands: reg means register, [mem] means memory location, and imm is an immediate operand. Also, x, y, and z denote the first, the second, and the third operand. Instruction mnemonics are written in capital letters to make them easier to find when you are skipping through the cheat sheet.
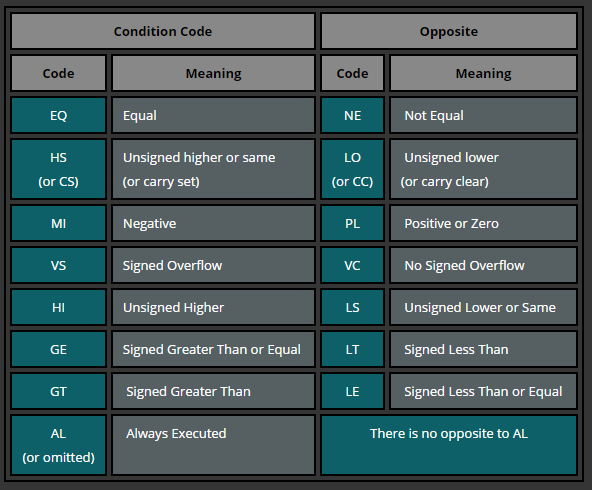
Example
For example, let's look at multiplication and division section. There are instructions for signed (IMUL) and unsigned (MUL) multiplication. Both instructions take one operand, which may be register (reg) or memory ([mem]). There are three possible cases:
- If operand size is one byte, MUL or IMUL multiplies it by al and stores the result in ax
- If operand size is a word, MUL or IMUL multiplies it by ax and stores the high-order word of the result in dx and the low-order word in ax.
- If operand size is a double word, MUL or IMUL multiplies it by eax and stores the high-order dword in edx and the low-order dword in eax.
There are also two-operand and three-operand forms of IMUL shown on the figure above.
Apple recommended mac cleaner. Other features of assembly language are described in a similar way.
Download
The cheat sheet is designed for A4 page size; if you print it on US Letter paper, you will get large margins. You can print the cheat sheet and put it on your table to look for some instructions when you forget them.
Serbo-Croatian translation of this article by WHG Team.
Command line options
Configuration properties
They can be used in evaluations:? ${asm.tabs}
You will want to set your favourite options in ~/.radare2rc
since every line there will be interpreted at the beginning of each session. Mine for reference:
There is an easier interface accessible from the Visual mode, just typing Ve
Basic Commands
Word 2016 download mac. Command syntax: [.][times][cmd][~grep][@[@iter]addr!size][|>pipe]
;
Command chaining: x 3;s+3;pi 3;s+3;pxo 4;
|
Pipe with shell commands: pd | less
!
Run shell commands: !cat /etc/passwd
!!
Escapes to shell, run command and pass output to radare buffer Note: The double exclamation mark tells radare to skip the plugin list to find an IO plugin handling this command to launch it directly to the shell. A single one will walk through the io plugin list.`
Radare commands: wx `!ragg2 -i exec`
~
grep~!
grep -v~[n]
grep by columns afl~[0]
~:n
grep by rows afl~:0
.cmd
Interprets command output
.
repeats last commands (same as enter n)(
Used to define and run macros$
Used to define alias$$
: Resolves to current address- Offsets (
@
) are absolute, we can use $$ for relative ones@ $$+4
?
Evaluate expression
?$?
Help for variables used in expressions$$
: Here$s
: File size$b
: Block size$l
: Opcode length$j
: When$$
is at ajmp
,$j
is the address where we are going to jump to$f
: Same forjmp
fail address$m
: Opcode memory reference (e.g. mov eax,[0x10] => 0x10)???
Help for?
command?i
Takes input from stdin. Eg?i username
??
Result from previous operations?s from to [step]
: Generates sequence fromto every ?p
: Get physical address for given virtual address?P
: Get virtual address for given physical one?v
Show hex value of math expr
?l str
: Returns the length of string@@
: Used for iterations
Positioning
Block size
The block size is the default view size for radare. All commands will work with this constraint, but you can always temporally change the block size just giving a numeric argument to the print commands for example (px 20)
JSON Output
Most of commands such as (i)nfo and (p)rint commands accept a j
to print their output in json
Analyze
Function analysis (normal mode)
Function analysis (visual mode)
Opcode analysis:
Information
Mitigations:
Get function address in GOT table:pd 1 @ sym.imp<funct>
Returns a jmp [addr]
where addr
is the address of function in the GOT. Similar to objdump -R | grep <func>
Write
Flags
Flags are labels for offsets. They can be grouped in namespaces as sym
for symbols ..
yank & paste
Visual Mode:
V
enters visual mode
ROP
Search depth can be configure with following properties:
Searching
Assembly Commands Cheat Sheet Download
Example: Searching function preludes:
Its possible to run a command for each hit. Use the cmd.hit
property:
Magic files
Search for magic numbers
Search can be controlled with following properties:
Yara
Yara can also be used for detecting file signatures to determine compiler types, shellcodes, protections and more.
Zignatures
Zignatures are useful when dealing with stripped binaries. We can take a non-stripped binary, run zignatures on it and apply it to a different binary that was compiled statically with the same libraries.
Zignatures are applied as comments:
Compare files
Graphs
Basic block graphs
Call graphs
Convert .dot in .png Macbook pro yosemite.
Generate graph for file:
Debugger
Start r2 in debugger mode. r2 will fork and attach
To pass arguments:
To pass stdin:
Commands
To follow child processes in forks (set-follow-fork-mode in gdb)
PEDA like details: drr;pd 10@-10;pxr 40@esp
Assembly Code Cheat Sheet
Debug in visual mode
WebGUI (Enyo)
All suite commands include a -r
flag to generate instructions for r2
rax2 - Base conversion
rahash2 - Entropy, hashes and checksums
Arm Assembly Cheat Sheet
radiff2 - File diffing
Examples:
rasm2 - Assembly/Disassembly
rafind2 - Search
ragg2 - Shellcode generator, C/opcode compiler
Example:
X86 Cheat Sheet
rabin2 - Executable analysis: symbols, imports, strings ..
Assembly Commands Cheat Sheet 2020
rarun2 - Launcher to run programs with different environments, args, stdin, permissions, fds
Arm Assembly Instructions Cheat Sheet
Examples:
